Are you looking for how to disable back button in flutter easily? You are in the right place. When users use your app, it is possible that they could unintentionally click the Back button and exit from the app. This can create a bad experience for your app. So it is always good to ask users if they are ready to exit the app. By default, from the home screen or app bar, if you press the back button you will exit from the app. So in this tutorial, we will show you that Disable Back Button in Flutter.
Table of Contents
The output will be looks like this:
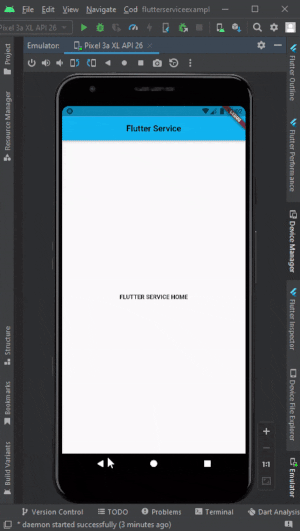
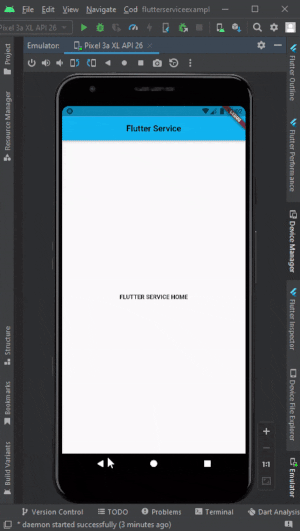
So what we will learn haaa? We will learn the following things from this article:
- How to Disable Back Button in Flutter?
- How to override and disable back button in Flutter?
How to Disable Back Button in Flutter
You can simply use the WillPopScope to disable the back button in Flutter. Now question is, what is WillPopScope? well, WillPopScope is a Flutter Widget that helps to get a callback when the back button is pressed.
So inside the WillPopScope callback, if you simply return false then the screen will not be popped, and the back button will be disabled, if you keep the callback return true then the screen will be popped.
Let’s see how can you do that:
- Wrap Scaffold widget by using the WillPopScope like this:
WillPopScope(
onWillPop: _onPop,
child: Scaffold(
…………………………………………………..
- Now add the _onPop parameter inside the WillPopScope. Then create a method and assign it to handle the callback.
- Now keep return false inside the callback.
Code Example:
class _MyHomePageState extends State<MyHomePage> {
Future<bool> _onPop() async {
return false;
}
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: _onPop,
child: Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(widget.title),
),
body: const Center(
child: Text(
"FLUTTER SERVICE HOME",
style: TextStyle(fontWeight: FontWeight.bold),
),
),
),
);
}
}
How to override and disable back button in Flutter?
You need to use the WilllPopScope widget to override that back button in flutter and then show the confirmation dialog to the user if they want to exit or not. So what will happen? If the user clicks the back button, it will show a dialog and ask a user for exit confirmation. If the user presses Yes then it will exit if the user presses No then it will not exit.
Same as before,
- Wrap Scaffold widget by using the WillPopScope like this:
WillPopScope(
onWillPop: _onWillPop,
child: Scaffold(
…………………………………………………..
- Now add the _onPop parameter inside the WillPopScope. Then create a method and assign it to handle the callback.
- Now add the AlertDialog widget inside the callback. Also, add options like Exit, Yes, or No using the TextButton or Elevated button what you like.
- Keep the Navigator.of(context).pop(false) inside the onPress() to stop exiting the app.
- Keep the Navigator.of(context).pop(true) inside the onPress() to exit the app.
Full Code Example:
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Future<bool> _onPop() async {
return (await showDialog(
context: context,
builder: (context) => AlertDialog(
title: new Text('Are you sure?'),
content: new Text('Exit the App'),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.of(context).pop(false),
//<-- SEE HERE
child: new Text('No'),
),
TextButton(
onPressed: () => Navigator.of(context).pop(true),
// <-- SEE HERE
child: new Text('Yes'),
),
],
),
)) ??
false;
}
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: _onPop,
child: Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(widget.title),
),
body: const Center(
child: Text(
"FLUTTER SERVICE HOME",
style: TextStyle(fontWeight: FontWeight.bold),
),
),
),
);
}
}
So we have learned how to override the back button / disable back button in flutter easily. We have seen how to disable the back button using the WillPopScope widget. After that, we have using the override the back button and display the user confirmation dialog. If you still face any issues, please send us a message from the chatbox. We are here to support you.
Read More: