This section will cover the idea of the flutter stateful widget setstate with parameters, the order in which states should be executed, and how to manage app states. Everything in Flutter is a widget, and there are two types of widgets based on their states. Two widgets,
- One stateful
- Stateless
The stateless widget has no states, and once it is created, there is no way to alter or change anything inside of it. But with a stateful widget, we can adjust both the widget’s appearance and its child structure as necessary. To create interactive apps, a flutter stateful widget setstate with parameters is crucial.
Table of Contents
Defining State
The widget’s state is a collection of data that is collected during construction and modification while an application is running. The setState() method can be used to update a widget’s state whenever you want to update or modify its structure. The user interface is updated, and the widgets are refreshed by setState() in response to recent state changes.
To create an interactive app, state management is a crucial step. All user interfaces are created by Flutter based on the declarations and app states that are currently in effect.
Create a Stateful Widget
You must extend the widget build class with “StatefulWidget” as shown below in order to create the stateful widget.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget{
@override
State createState() {
return _HomePage();
}
}
class _HomePage extends State{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar( //appbar widget inside Scaffold
title: Text("Stateful Widget")
),
body: Center(
child: Text("Stateful Widget"),
)
);
}
}
The HomePage widget in the code above has a child class named _HomePage() that extends the state of its parent class.
How to update the State and Widgets in the App
You might need to modify the data and user interface (UI) in an interactive and dynamic app depending on the situation. Although widgets are updated using the setState() method, variables’ values, and states may change in the background. See the example below to demonstrate how to update the state and widgets within an application. A floating action button and a centered text with a counter value can be seen in the example code that follows. The counter value will be increased when the floating action button is pressed, and setState() will be used to update the widget’s displayed value.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget{
@override
State createState() {
return _HomePage();
}
}
class _HomePage extends State{
int counter;
@override
void initState() { //inital state which is executed only on initial widget build.
counter = 0;
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar( //appbar widget inside Scaffold
title: Text("Stateful Widget")
),
floatingActionButton: FloatingActionButton(
onPressed: (){
setState(() { // setState to update the UI
counter++; //during update to UI, increase counter value
});
},
child: Icon(Icons.add), //add icon on Floating button
),
body: Center(
child: Text("Counter: $counter"), //display counter value
)
);
}
}
Output:
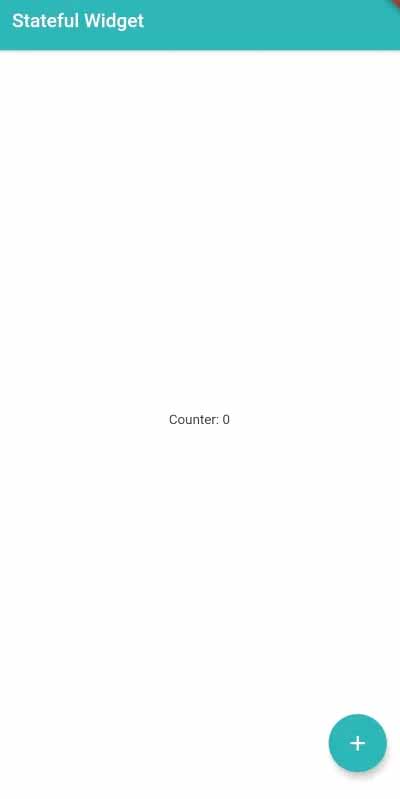
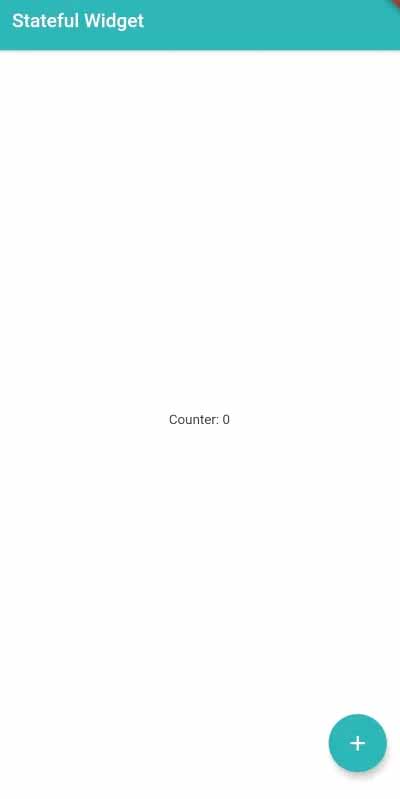
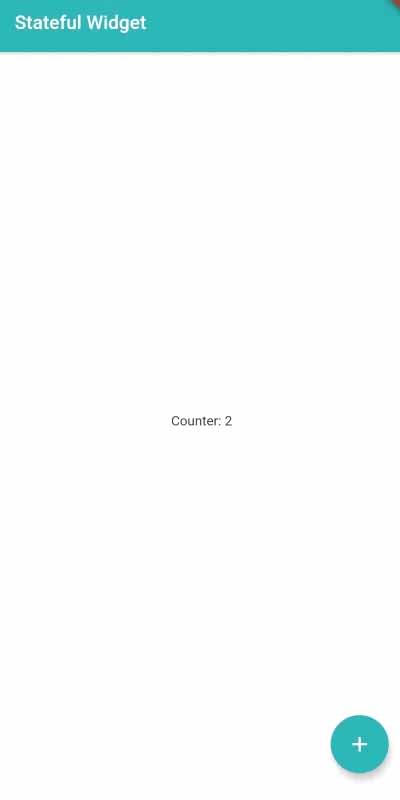
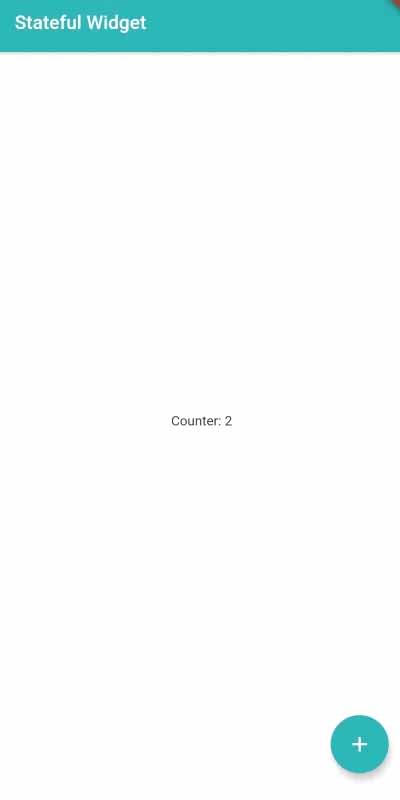
In-App Execution Order for flutter stateful widget setstate with parameters:
If you’re developing an app, it’s essential to comprehend the code execution flow. To understand the execution flow in Flutter, look at the example code below and its result.
import 'package:flutter/material.dart';
void main() {
print("1. main method executed.");
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
print("2. MyApp widget build.");
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget{
@override
State createState() {
print("3. HomePage state build.");
return _HomePage();
}
}
class _HomePage extends State{
int counter;
@override
void initState() { //inital state which is executed only on initial widget build.
print("4. Initial state of _HomePage.");
counter = 0;
super.initState();
}
@override
Widget build(BuildContext context) {
print("5. Widget build of _HomePage.");
return Scaffold(
appBar: AppBar( //appbar widget inside Scaffold
title: Text("Stateful Widget")
),
floatingActionButton: FloatingActionButton(
onPressed: (){
print("----button pressed----");
setState(() { // setState to update the UI
print("6. setState() after button press.");
counter++; //during update to UI, increase counter value
});
},
child: Icon(Icons.add), //add icon on Floating button
),
body: Center(
child: Text("Counter: $counter"), //display counter value
)
);
}
}
Output:
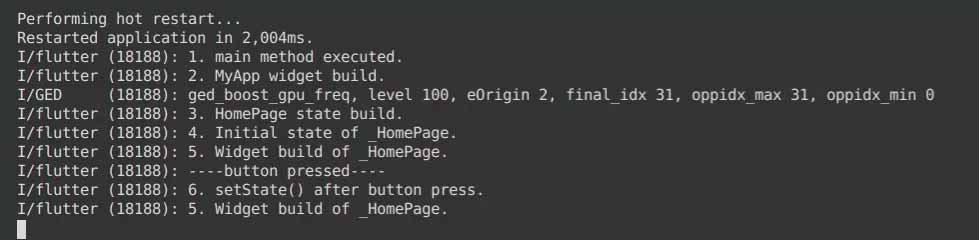
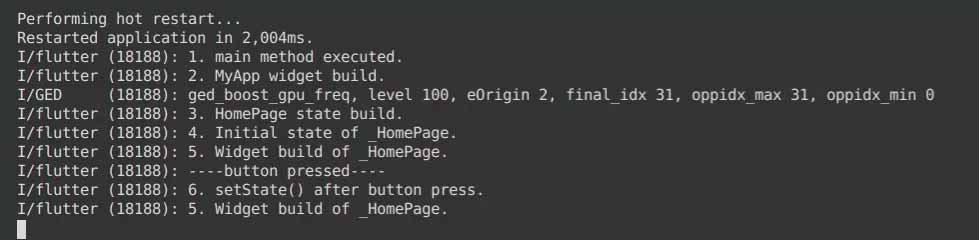
You must make a note of the following two points from the output above.
- initState() is executed only one time before the widget build.
- Widget build repeats after setState().
People, who read this article also read: Flutter Liquid Swipe Animation
Conclusion
You’ve learned the basics of how to use flutter stateful widget setstate with parameters.
Now, I’d like to hear from you: Do you know how to use the flutter stateful widget setstate with parameters? Did you think it would be this easy?
Either way, let me know by leaving a quick comment below.