Gesturedetector flutter example are movements and actions performed physically on an app’s screen with the intention of commanding or controlling the app. The capability to communicate with users is crucial. The following are a few gesture examples:
- Screen lock sliding.
- Tap the screen of a mobile device.
- Holding on to the button with your finger.
Table of Contents
Introduction
A built-in mechanism for detecting gestures is not present in some widgets, such as the Container and Card widgets. These widgets are encased in the GestureDetector flutter example widget, which is solely used for gesture detection and does not produce any visual effects like a ripple.
The GestureDetector flutter widget functions by identifying gestures for which callbacks have been defined and reacting to the event. The callback receives a null value if the gesture is to be disabled.
The common gestures detected by the GestureDetector widget are listed below, along with the corresponding events and potential applications (all illustrations are credited to Luke Wroblewski’s Touch Gesture Reference Guide):
Scale
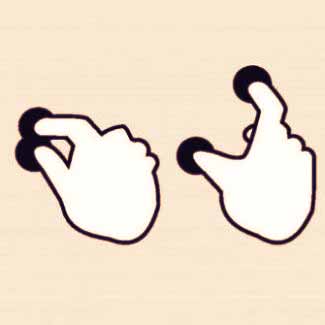
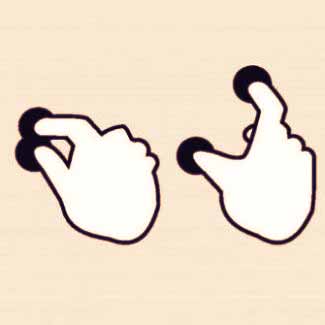
- onScaleUpdate — When the user is not in contact with the screen anymore, onScaleEnd is triggered. nd/or scale
- onScaleEnd — triggered once the user has lost contact with the screen Possible application for the scale gesture
- onScaleStart — triggered when the screen has made contact with the user, establishing a focal point and an initial scale of 1.0.
Scale gestures have the following uses:
- Rotation
- Vertical Drag
- Zoom in/zoom out
Double Tap
Twice in a short period of time, the user tapped the screen in the same place.
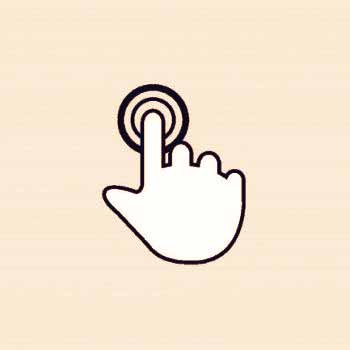
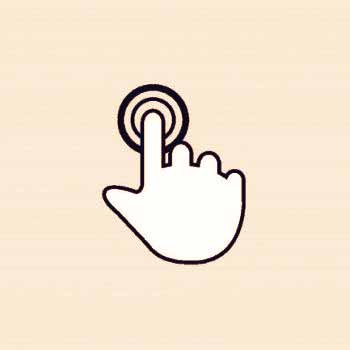
- onDoubleTapDown — triggered when a user touches the screen, possibly with a double tap
- onDoubleTapCancel — triggered if the event that fired onDoubleTapDown is not a double tap.
- onDoubleTap — triggered whenever a user quickly and repeatedly taps the same spot on the screen twice.
People, who read this article also read: Flutter Liquid Swipe Animation
The following examples illustrate possible uses for the double-tap gesture:
- Long press
- Like/dislike
- Resize an image
- Screen on/off
Long Press
For a considerable amount of time, the user maintained contact with the screen at the same point.
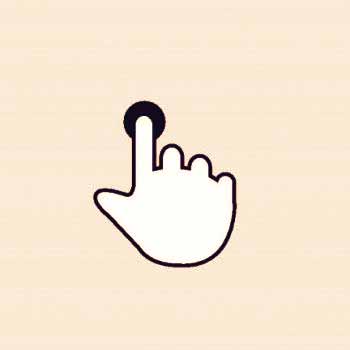
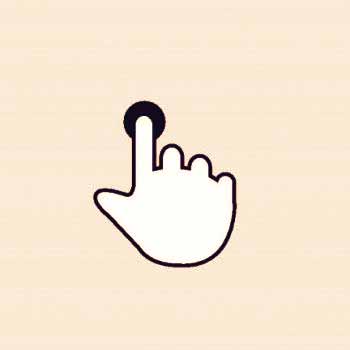
- onLongPressCancel — When the event that fired onLongPressDown is not a long press, the trigger occurs.
- onLongPressStart — triggered when the beginning of a long press is discovered
- onLongPress — triggered after the detection of a long press
- onLongPressEnd — triggered when the conclusion of a long press is discovered
- onLongPressDown — triggered when a user touches the screen; this contact may be prolonged
- onLongPressMoveUpdate — triggered when a long press has been detected and the user has moved their finger.
- onLongPressUp — triggered when the end of a long press is discovered; contact has been released following the long press
The following are some potential uses for the long-press gesture:
- The user pinched or spread the screen.
- Move an icon
- Scale
- Show more options
Tap
A fingertip of the user made brief contact with the screen.
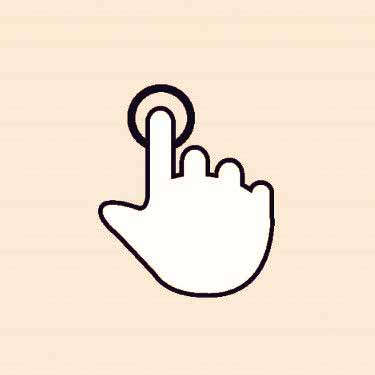
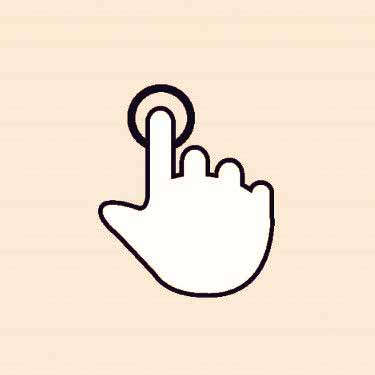
- onTapUp — triggered when the user removes their eyes from the screen
- onTapDown — triggered when the user makes physical contact with the screen, which could be a tap.
- onTapCancel — triggered if the event that fired on TapDown is not a tap
- onTap — triggered when a user only fleetingly touches the screen
The following are some potential uses for the tap gesture:
- Cancel
- Double-tap
- Select
- Submit
Vertical Drag
The user touched the screen and steadily moved their fingertip up and down.
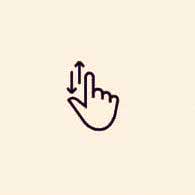
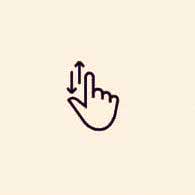
- onVerticalDragStart — the user begins to move vertically after making contact with the screen, which triggers the action.
- onVerticalDragDown — it may move vertically when a user touches the screen and is triggered by that touch.
- onVerticalDragEnd — It starts when the conclusion of a vertical drag is found.
- onVerticalDragCancel — triggered if the event that caused VerticalDragDown to fire is not a vertical drag.
- onVerticalDragUpdate — triggered when a contact that has been moving vertically stops and starts again.
The vertical drag gesture may be used for the following purposes:
- Scroll
Horizontal Drag
A steady horizontal movement was made by the user’s fingertip after making contact with the screen.
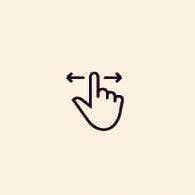
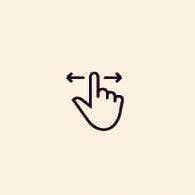
- onHorizontalDragStart — triggered as soon as the user starts moving horizontally after making contact with the screen.
- onHorizontalDragEnd — when a horizontal drag has ended and the end has been detected.
- onHorizontalDragCancel — occurs when an event that fired on HorizontalDragDown is not a horizontal drag.
- onHorizontalDragDown — when the user touches the screen, a movement may begin that could be horizontal.
- onHorizontalDragUpdate — triggered when a horizontally moving contact moves in a horizontal direction once more.
The following are some potential uses for the horizontal drag gesture:
- Go to a different view by navigating.
- Delete
- Archive
This is not an exhaustive list of the gestures that were discovered. For a full list, refer to the official documentation.
Let’s give it a shot!
Starting Up
To use the GestureDetector flutter example widget:
- Give a callback for the gesture you want to recognize.
- This app needs to be updated.
- Wrap the GestureDetector widget around the desired widget.
To demonstrate how the tap, double-tap, long press, and scale gestures work, we will create a straightforward demo app.
Make a fresh Flutter application
Clear the default code from your main.dart file before creating a new Flutter application.
UI update
These four files will be made by us. The folder hierarchy is displayed here.
main.dart
import 'package:flutter/material.dart';
import 'presentation/my_app_widget.dart';
void main() {
runApp(const MyApp());
}
My_app_widget.dart
import 'package:flutter/material.dart';
import 'home_page.dart';
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Gesture Detector Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
Home_page.dart
import 'package:flutter/material.dart';
import 'widgets/widgets.dart';
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
final height = MediaQuery.of(context).size.height;
final width = MediaQuery.of(context).size.width;
return Scaffold(
body: Padding(
padding: EdgeInsets.symmetric(
horizontal: width * 0.1, vertical: height * 0.2),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: const [
MyCardWidget(),
MyFavoriteIconWidget()
],
),
),
);
}
}
My_card_widget.dart
import 'dart:math';
import 'package:flutter/material.dart';
class MyCardWidget extends StatefulWidget {
const MyCardWidget({
Key? key,
}) : super(key: key);
@override
State<MyCardWidget> createState() => _MyCardWidgetState();
}
class _MyCardWidgetState extends State<MyCardWidget> {
@override
Widget build(BuildContext context) {
return const Card(
child: SizedBox(
height: 300,
width: 300,
),
color: Colors.yellow,
);
}
}
My_favorite_icon_widget.dart
import 'package:flutter/material.dart';
class MyFavoriteIconWidget extends StatefulWidget {
const MyFavoriteIconWidget({
Key? key,
}) : super(key: key);
@override
State<MyFavoriteIconWidget> createState() => _MyFavoriteIconWidgetState();
}
class _MyFavoriteIconWidgetState extends State<MyFavoriteIconWidget> {
@override
Widget build(BuildContext context) {
return const Icon(
Icons.favorite_border,
size: 40,
);
}
}
Your completed app should resemble this:
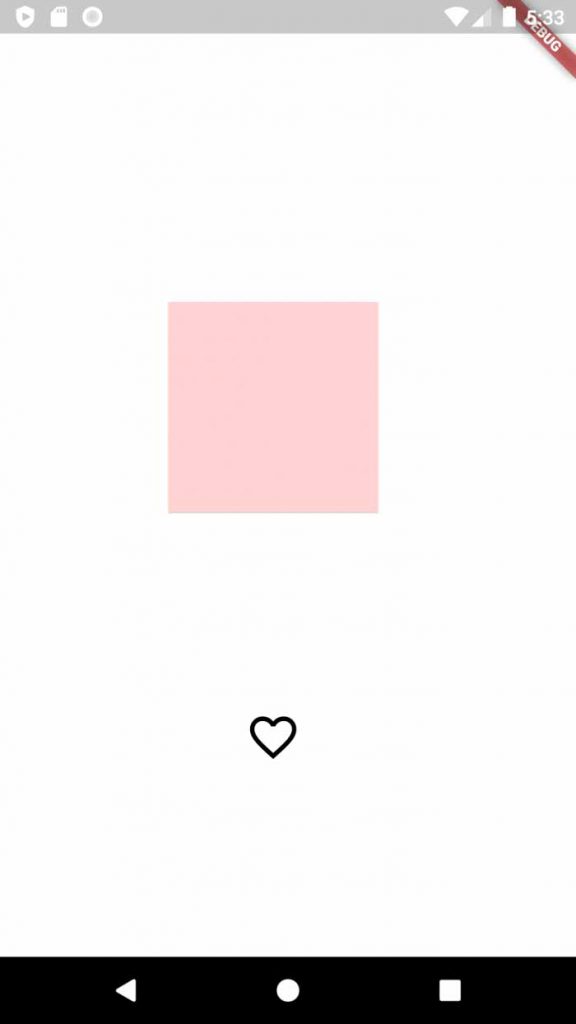
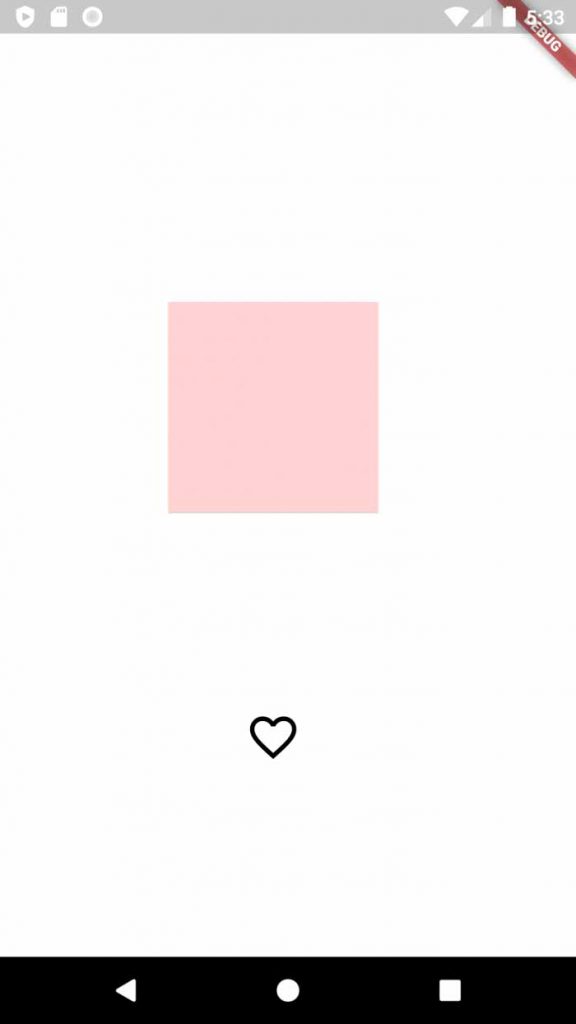
Let’s handle some gestures now that our user interface is prepared.
Managing the Tap Gesture
Now, in the my_favorite_icon_widget.dart file:
- Make the StatefulWidget’s selected flag property available.
- isSelected = false in the bool;
- Wrap the GestureDetector widget around the Icon widget.
- Don’t leave the onTap property’s callback as null.
- Depending on the value of the flag property value, alter the icon and icon color.
class _MyFavoriteIconWidgetState extends State<MyFavoriteIconWidget> {
bool isSelected = false;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: (){
setState(() {
isSelected = !isSelected;
});
},
child: Icon(
isSelected ? Icons.favorite: Icons.favorite_border,
size: 40,
color: isSelected? Colors.red: Colors.black ,
));
}
}
Managing the Double-Tap Gesture
In the my_card_widget.dart file:
- Include a color property.
- GestureDetector should be wrapped around the Card widget.
- Give the onDoubleTap property a non-null callback.
- Based on the value of the color property, alter the color of the card.
class _MyCardWidgetState extends State<MyCardWidget> {
Color bgColor = Colors.yellow;
@override
Widget build(BuildContext context) {
return GestureDetector(
onDoubleTap: (){
setState(() {
bgColor = Colors.primaries[Random().nextInt(Colors.primaries.length)];
});
},
child: Card(
child: const SizedBox(
height: 300,
width: 300,
),
color: bgColor,
),
);
}
}
Managing the Long Press Gesture
In the my_card_widget.dart file:
- Give the onLongPress property a non-null callback.
- Depending on the makeCircular property, alter the card’s shape.
- Add a makeCircular flag property
class _MyCardWidgetState extends State<MyCardWidget> {
Color bgColor = Colors.yellow;
bool makeCircular = false;
@override
Widget build(BuildContext context) {
return GestureDetector(
onLongPress: (){
setState(() {
makeCircular = !makeCircular;
});
},
child: Card(
shape: makeCircular? const CircleBorder(): const RoundedRectangleBorder(),
child: const SizedBox(
height: 300,
width: 300,
),
color: bgColor,
),
);
}
}
Managing the Scale Gesture
In the my_card_widget.dart file:
- Insert the _scaleFactor property.
- Include the _baseFactor property.
- Establish an initial scale by providing a non-null callback to the onScaleStart property.
- Establish a new scale by providing a non-null callback to the onScaleUpdate property.
- Return to the initial scale by providing a non-null callback to the onScaleEnd property.
- Transorm.scale widget should encircle the Card widget.
- Depending on the _scaleFactor’s value, modify the scale property.
class _MyCardWidgetState extends State<MyCardWidget> {
Color bgColor = Colors.yellow;
bool makeCircular = false;
double _scaleFactor = 0.5;
double _baseScaleFactor = 0.5;
@override
Widget build(BuildContext context) {
return GestureDetector(
onScaleStart: (details){
_baseScaleFactor = _scaleFactor;
},
onScaleUpdate: (details){
setState(() {
_scaleFactor = _baseScaleFactor * details.scale;
});
},
onScaleEnd: (details){
// return to initial scale
_scaleFactor = _baseScaleFactor;
},
child: Transform.scale(
scale: _scaleFactor,
child: Card(
shape: makeCircular? const CircleBorder(): const RoundedRectangleBorde(),
child: const SizedBox(
height: 300,
width: 300,
),
color: bgColor,
),
);
}
}
The following video demonstrates the gestures used:
Gesture Clarification
What transpires then when two delayed, fleeting touch events take place when we provide the onGestureDown event callback for tapping and double-taping?
Take this as an example:
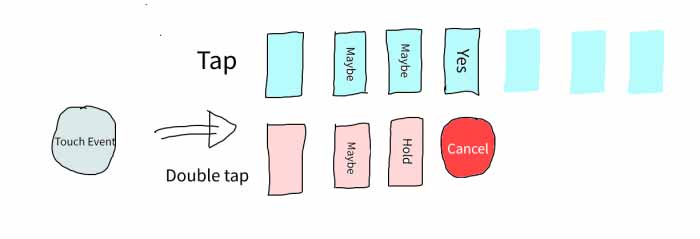
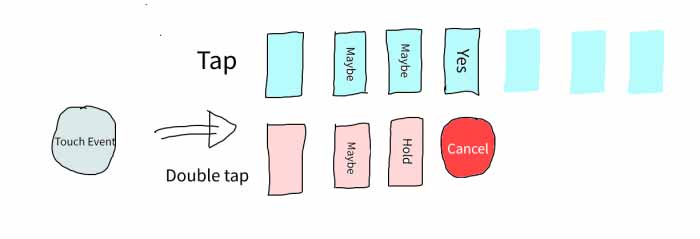
Flutter determines which gesture the user intended when two or more gesture events with non-null callbacks are recognized by having each recognizer enter the gesture arena. The winning event in the gesture arena “battles” with the closing events, which are canceled.
People, who read this article also read: Flutter SDK Installation on Windows, macOS, Linux
The following factors are considered by the gesture arena:
- The amount of time a user touches a screen
- How many pixels were moved in each direction
- What movement is present in the room?
- A move that signals success
The battle states are as follows:
- Maybe — possibly the gesture
- Hold — if it changes in a specific way; in our case, one tap happened, and if the second tap happens within the anticipated time, it might be a double tap. might be the gesture if it develops in a specific way.
- Yes — announcement of victory
- Cancel — removed from combat
Consider the following scenario, for instance:
- The triggers for onTapDown and onDoubleTapDown
- Both gestures are in competition with one another.
- The onTap callback is carried out after the tap gesture succeeds.
- OnDoubleTapCancel is triggered when the double-tap gesture fails and is canceled.
In this instance, the tap gesture prevailed because:
- There was a delay in time between the two taps.
- With a tap and a “yes,” victory was declared.
- After the double-tap was canceled and there was no other competitor, the tap gesture was the only one left.
Conclusion
We have examined the GestureDetector widget and discovered how it functions. We have mastered its use to incorporate interactivity into our application, and we have put some of the standard gestures—such as tap, long press, double-tap, and scale—into practice. Finally, we considered gesture classification.