This is a guide to getting started with Flutter Hello World. You can finish this tutorial if you are familiar with object-oriented programming and fundamental programming ideas like variables, loops, and conditionals. No prior knowledge of Dart, mobile, desktop, or web programming is required.
Everything in Flutter is a widget, and using predefined widgets, one can create custom widgets just like one can create custom data types using int, float, and double. Three widget types can be found in Flutter.
- Stateless Widget
- Stateful Widget
- Inherited Widget
Table of Contents
What You Plan To Construct
You’ll implement a simple app that generates “Hello World”
In this article, we will use Stateless Widget, Stateful Widget, center, material app, and Inherited Widget.
Stateless Widget: In Flutter, stateless widgets are those that cannot be changed. To draw components on the screen, stateless widgets have a method (or function) called build that is called just once. A stateless widget must be created in a new instance in order to be redrawn.
MaterialApp: This widget is also offered by the Flutter Team and adheres to the Google Material Design Scheme. MaterialApp is a class with a number of named arguments, such as home, where we can pass the widget that will be displayed on an app’s home screen.
Flutter Documentation has more information about MaterialApp.
People, who read this article also read: Flutter SDK Installation on Windows, macOS, Linux
Center Widget: The Flutter Team has also predefined the Center widget, which accepts another widget as its child argument. As the name implies, Center Widget will display Widget in its child argument.
Center(
child: Widget(
),
),
Getting Started with Flutter Hello World
Flutter apps can also be built for desktops. Your operating system should be listed under devices in your IDE, such as Windows (desktop), or it may be specified at the command line using flutter devices.
Text Widget: The Flutter Team has also predefined a text widget that is used to display text. Let’s create a Hello World app with Flutter now.
The package that includes definitions for Stateless Widget, Center, Text, Material App, and many more are being imported at this time. Similar to a C++ program’s #include<iostream> statement.
Build: This method is in charge of painting components on the screen. It accepts a BuildContext argument that specifies which widgets should be painted on the screen first and in what order.
To create the “Hello World” app, open the main.dart file, remove all default codes, and then follow the instructions below.
import 'package:flutter/material.dart';
void main() {
// initial default method which is first executed
}
The first method that will automatically run is main(). You must begin writing more code inside of this method.
Make a new class now and add StatelessWidget as an extension.
import 'package:flutter/material.dart';
void main() {
}
class MyApp extends StatelessWidget{
}
The following code will appear when you click the yellow balloon that appears after you click the red line that appears on “MyApp” in VS Code or Android Studio because of a syntax error.
import 'package:flutter/material.dart';
void main() {
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
// TODO: implement build
throw UnimplementedError();
}
}
Put the code below in the main() method.
runApp(MyApp());
Create a class for the home page now and extend it with StatelessWidget.
class Home extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Welcome to Flutter")
),
body: Center(
child: Text("Hello World"),
)
);
}
}
Put the following code in the widget build of the MyApp class now:
return MaterialApp(
home: Home(), //home class
);
Final Code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
);
}
}
class Home extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Welcome to Flutter")
),
body: Center(
child: Text("Hello World"),
)
);
}
}
This is how you can be getting started with flutter “Hello World” on your first application, on a real mobile device, or on an emulator right away. The final product will resemble the pictures below.
Output
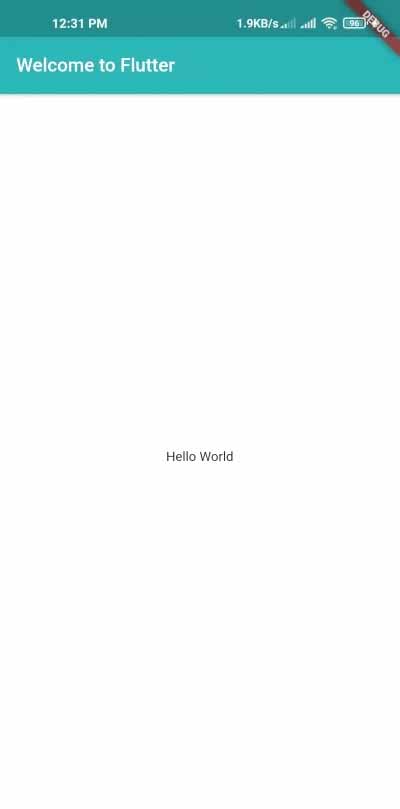
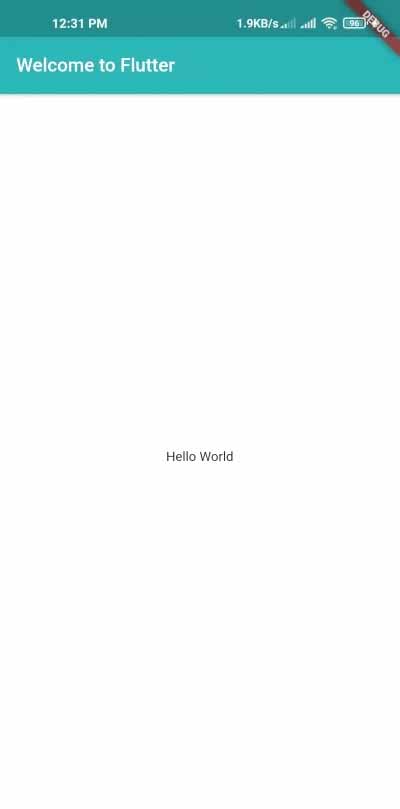
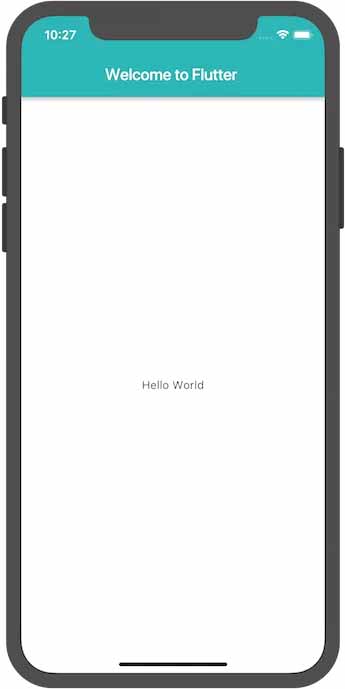
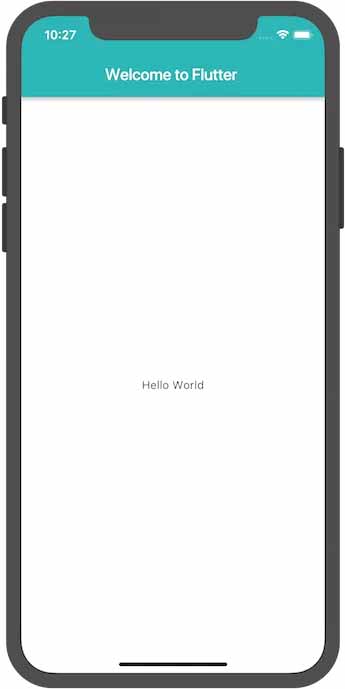
Analysis of the output
The first line imports the material design library that will be used in this application. Then comes our primary purpose. The execution of the code will begin at this point.
- This is essentially our widget tree for getting started with flutter “hello world” app.
- The build method, which returns a MaterialApp widget, comes after all of this.
- Next, we used MaterialApp’s home property, which holds the Scaffold widget. The entire app screen is contained by the Scaffold widget.
- We have made use of the AppBar property, which accepts the AppBar widget as an object.
- The AppBar widget then has the title “GFG” displayed. The body is the following item, which is once more a MaterialApp property.
- The text widget that says “Hello World” is a child of the Center, which is the body’s object.
A word of advice: Indentation may become distorted when pasting code into your app. The formatting tool listed below can be used to fix this:
- VS Code: Right-click and choose “Format Document” in VS Code.
- Java IDEA and Android Studio: Reformat the code with dartfmt by right-clicking it. Run the command flutter format in the terminal.
Observations
This example shows how to make a Material app. Material is a visual design language that is widely used on mobile devices and the web. Flutter provides a diverse set of Material widgets. It’s a good idea to include a uses-material-design: true entry in the flutter section of your pubspec.yaml file. This enables you to use more Material features, such as its predefined Icons.
- The app extends StatelessWidget, making it a widget in and of itself. Alignment, padding, and layout are all widgets in Flutter.
- The Scaffold widget from the Material library includes a default app bar and a body property that holds the home screen widget tree. The widget subtree can be quite complex.
- The primary function of a widget is to provide a build() method that describes how to display the widget in terms of other, lower-level widgets.
- The body for this example consists of a Center widget containing a Text child widget. The Center widget aligns its widget subtree to the center of the screen.
People, who read this article also read: Flutter Liquid Swipe Animation
Conclusion of Getting Started With Flutter Hello World
You can compile each Flutter app you make for the web as well. Currently, Chrome and the Web server should be listed in your IDE’s devices pull-down menu or at the command line using flutter devices. Chrome launches by default on the Chrome device. To enable you to load the app from any browser, the Web server launches a server that hosts it. Use the Chrome device while developing in order to access DevTools and the web server for testing on different browsers. More details are available in the articles on getting started with flutter hello world on the web.
Check this video for further details about Getting Started With Flutter Hello World: