Flutter SizedBox widget is what we know as a box with a specific size. Now, let’s learn it in depth.
Table of Contents
What is Flutter SizedBox?
Flutter SizedBox, unlike Container, does not support color or decoration. The widget is only used to resize the widget supplied as a parameter. The default constructor and certain named constructors are used in the examples below.
How the Flutter SizedBox help to Align
It’s a box widget in which we specify the size of the box.
- If the child widget has no height or width, we must provide the SizedBox widget the dimension.
- Now, If we leave the height and width parameters blank, it will use the child’s height and width as its dimensions. If the child’s size is determined by its parent’s size, the height and width must be specified.
- SizedBox will try to size itself as near to the supplied height and width as feasible given the parent’s limitations if it is not given a child. If the height or width is null or undefined, it is assumed to be zero.
If the width or height are both null, this widget will try to scale itself to match the child’s size in that dimension.
People, who read this article also read: Flutter LayoutBuilder Widget Example Step by Step
SizedBox Widget has the following properties:
- child: This property accepts a child widget as an object and displays it beneath the SizedBox in the widget tree or within the SizedBox on the screen.
- height: The height of SizedBox in pixels is specified by this attribute. It has the same value as the object.
- width: This property likewise has a double value as the object to provide the SizedBox width.
- key: This key is for the widget.
Flutter SizedBox Vs. Container
Containers may also be utilized to provide separation between widgets. Alternatively, cover the child and give it a certain height.
However, Container has several unique qualities.
So, instead, we may use SizedBox, which allows us to define the child, height, and width. However, it functions similarly.
Also, because Container has more attributes, it is a heavier widget, and you may make the SizedBox widget constant. This improves efficiency.
Why is the Flutter SizedBox in use?
This widget, when given a child, compels it to have a particular width and/or height. If the parent of this widget does not allow them, these settings will be ignored. This occurs, for example, when the parent is a screen (which compels the child to be the same size as the parent) or another SizedBox (which forces its child to have a specified width and/or height). This may be fixed by enclosing the child SizedBox in a widget that allows it to be any size up to the parent’s size, such as Center or Align.
The Expand Constructor SizedBox
The expand constructor may be used to create a SizedBox that expands to accommodate the parent. It is the same as doubling the width and height.
How to Make Use of the SizedBox Widget
- Allow space between widgets
- Modify the Widget Size
Allow space between widgets.
As previously stated, SizedBox is utilized to provide space between widgets.
const SizedBox(height: 20), //Add space Vertically
const SizedBox(width: 20), //Add Space Horizontally
Modify the Widget Size
We specify the SizedBox’s height and width. It will disregard the child widget’s width and height and give it a custom width and height.
SizedBox(
height: 20, //This become the height of the container
child: Container(
height: 100, //This height will be ignored
color: Colors.red,
),
),
Output:
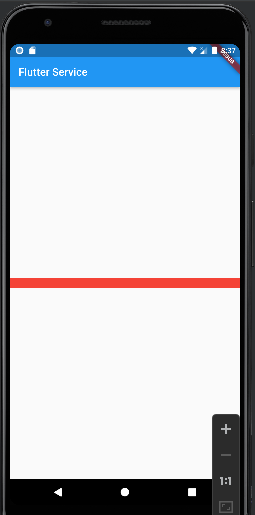
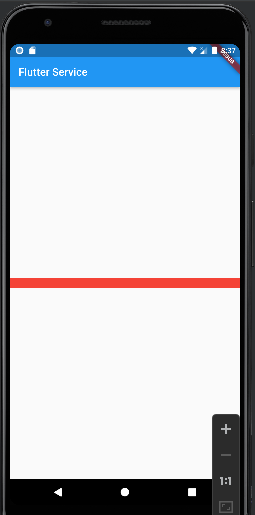
If we use double.infinity() as the height or width of the flutter SizedBox widget, it will occupy the parent widget’s whole width or height.
Scaffold(
body: SizedBox(
height: double.infinity, // This will take the whole space
width: double.infinity,
child: Container(
height: 100,
color: Colors.red,
),
),
);
Output:
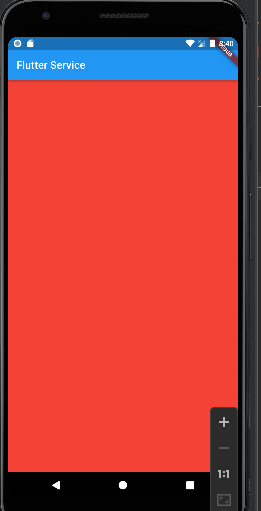
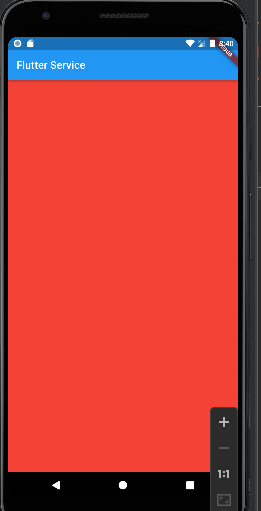
Constructors of SizedBox
Name constructors for SizedBox are as follows:
- SizedBox.fromSize()
- SizedBox.expand()
- SizedBox.shrink()
SizedBox.fromSize()
The Size() object is used to create a SizedBox widget. The Size() Object will be sent to this constructor.
SizedBox.fromSize(
size: const Size(100, 150), //(width, height)
child: Container(
height: 100,
color: Colors.red,
),
),
Output:
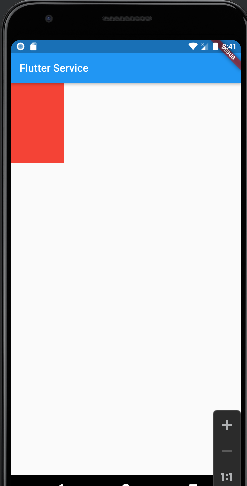
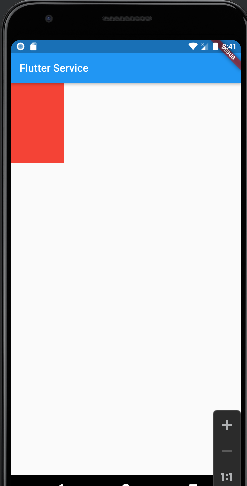
What exactly is Size()?
It’s from the dart user interface. When working with media queries. The size of the device may be obtained from the media query by utilizing.
We may also pass width and height through it.
Size() width and height are therefore used by the SizedBox widget to generate flutter UI.
Instead of passing the height and width to the SizedBox, we now use Size ().
People, who read this article also read: How to Use the Flutter Positioned Widget?
Where SizedBox.fromSize() comes in handy.
SizedBox.expand()
This constructor can also be used to expand the child to an unlimited width and height, allowing it to fill all available space.
Scaffold(
body: SizedBox.expand( //Behave just like double.infinity height and width
child: Container(
height: 100,
color: Colors.red,
),
),
);
SizedBox inside the Column Widget
When we set the double.infinity width to the SizedBox inside the Column widget. It will take up the entire available screen area.
Height and width are both infinite
Column(
children: [
SizedBox(
width: double.infinity, // Take whole horizontal space
child: Container(
height: 100,
color: Colors.red,
),
),
],
),
Output:
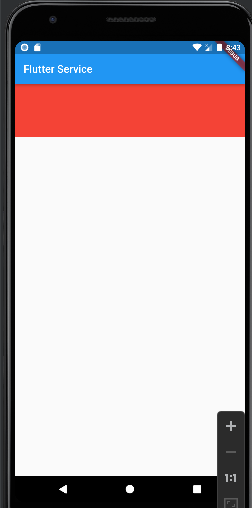
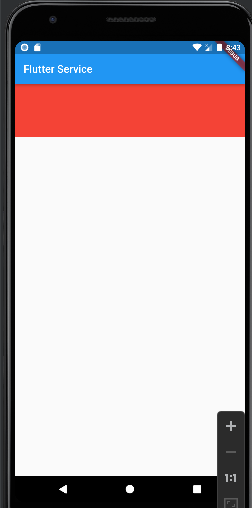
Exception Error
However, if we set the double.infinity to the SizedBox widget’s height property. It will not occupy the entire vertical area on the screen. Keep it in mind! (In this case, Column BoxConstraints compels an unlimited height.)
Solution
Simply utilize the Expanded widget to Make SizedBox to take up the whole height of the screen.
Previously
Column(
children: [
SizedBox(
height: double.infinity, //Inside column this will produce error
child: Container(
height: 100,
color: Colors.red,
),
),
],
),
Now
Column(
children: [
Expanded( //This will solve the problem
child: SizedBox(
height: double.infinity,
child: Container(
height: 100,
color: Colors.red,
),
),
),
],
),
SizedBox.shrink()
This constructor serves to reduce the size of everything inside the SizedBox. But the question is, how can we put it to use?
SizedBox.shrink(
child: Container(
height: 300,
color: Colors.red,
),
),
We utilize SizedBox with ConstrainedBox widgets constraints property and assign BoxConstraints to determine the minimum height and width.
Invisible Object SizedBox
SizedBox is invisible by default. That is why we use it to represent space. Consider Container Widget if you wish to colorize the box.
Padding with SizedBox
Consider the following example.
AppBar(
title: const Text('MY PAGE'),
actions: [
IconButton(
onPressed: () {},
icon: const Icon(Icons.search),
),
const SizedBox(
width: 20, //Here Sized Box will add some padding after search icon
)
],
)
Output:
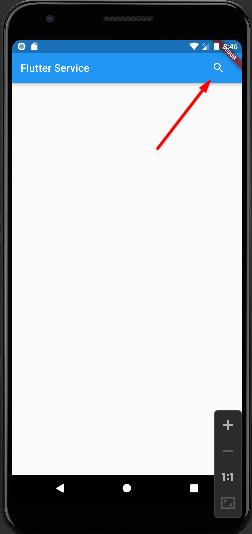
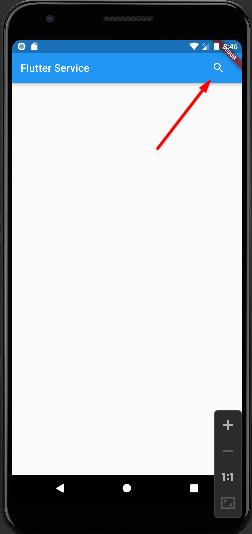
ListView with Flutter SizedBox
Let’s look at how SizedBox may increase space to a ListView.
ListView(
children: const [
Text('First Widget'),
SizedBox(height: 20),
Text('Second Widget')
],
),
Row Widget with Flutter SizedBox
SizedBox may also serve inside the Row widget as well.
Row(
children: const [
Text('First Widget'),
SizedBox(width: 20), //Add horizontal space
Text('Second Widget')
],
),
Sized Boxes in Flutter
There are additional widgets in Flutter that have SizedBox as part of their name.
- FractionallySizedBox – A fraction of the total available space is used to size its child.
- SizedOverflowBox – a specified size widget whose limitations are passed on to its child.
Make a Full-Width Button with SizedBox
Let’s construct a raised button with the same width as the screen.
Column(
children: [
SizedBox(
width: double.infinity,
child: ElevatedButton(
onPressed: () {}, child: const Text('My Button')))
],
),
Output:
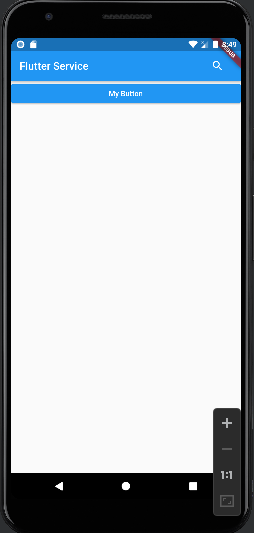
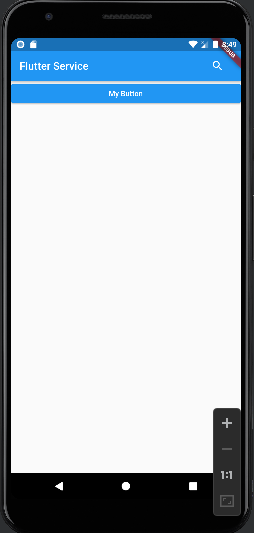
Conclusion
You’ve learned the basics of how to utilize Flutter SizedBox.
Now, I’d like to hear from you: Do you know how to use the flutter SizedBox attribute? Did you think it would be this easy?
Either way, let me know by leaving a quick comment below
In this Flutter Tutorial, we learned about the SizedBox and how to use it with examples from various circumstances.