For loop is the most commonly used loop in programming languages. If you want to know How to Use For Loop in Flutter then you are in the right place. We will discuss the best practice for using the For Loop in Flutter.
We know that For loop is a loop that enables a particular set of conditions that will be executed repeatedly until a condition is satisfied. So for loop will execute the code a specific number of times. We know that for loop is the very basic loop in all programming languages.
What we will learn from this article:
Table of Contents
Syntax of for loop in Flutter
for (initial_count_value; termination_condition; step) {
// statements
}
Example of for loop
1st Example:
If you don’t need the index then you can consider the for-in loop. It’s very easy to read and more concise.
List<String> fruits= ['Apple', 'Banana', 'Mango'];
for (var fruit in fruits) {
print(fruit );
}
// prints
'Apple'
'Banana'
'Mango'
2nd Example:
This example will provide you the index of the looping position.
void main() {
for(var j = 0; j < 4; j++) {
print(j);
}
}
//prints
0
1
2
3
You also can use the loop on the widget to create a list of widgets. Let’s Use this For Loop in Flutter. You can see the output will be shown with the index number.
Full Code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.lightBlue,
),
home: const MyHomePage(title: 'Flutter Service'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Future<bool> _onPop() async {
return false;
}
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: _onPop,
child: Scaffold(
appBar: AppBar(
centerTitle: true,
title: Text(widget.title),
),
body: Center(
child: Column(
children: [
// you can add any widget here
// below we use for loop
for (int i = 0; i < 4; i++) ...{
Card(
child: Container(
width: double.infinity,
padding: EdgeInsets.all(20),
child: Text("Item List - $i")),
)
}
],
),
),
),
);
}
}
Output
The output of this For Loop in Flutter will look like this:
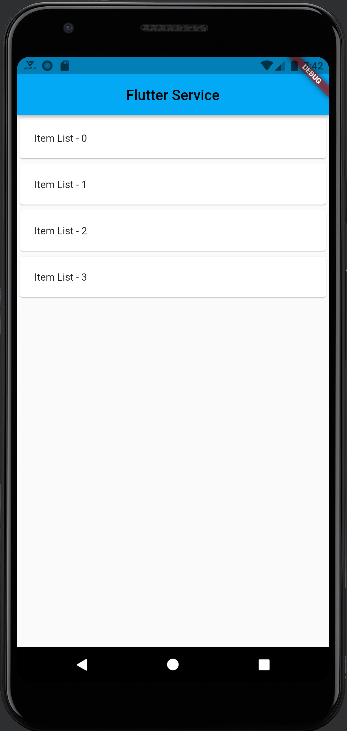
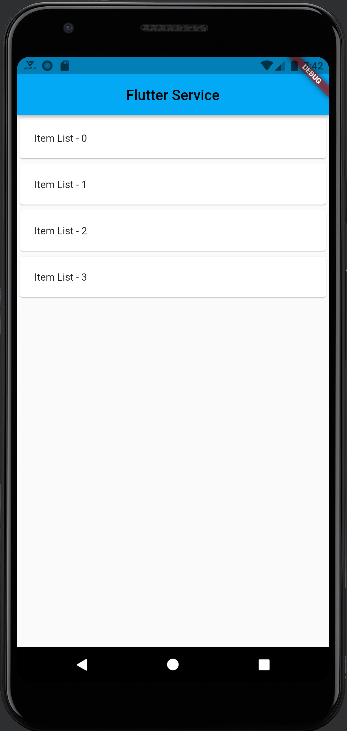
This is how we can use the for loop in flutter with widgets children.
Read More: