Path Provider Flutter is a Flutter package for finding commonly used locations on the filesystem. Path Provide Flutter is an important package in Flutter for working with files and directories on the file system. Path Provide Flutter allows developers to easily access commonly used locations such as the temporary directory and the application documents directory, which are the locations where files are typically stored. These locations can be used to read or write files in Flutter, which is important for many applications that need to save and retrieve data.
For example, an application that allows users to take photos and save them to their device will need to use the Path Provider flutter to determine the location to save the photos. Similarly, an application that allows users to create and save documents will need to use the Path Provider flutter to determine the location to save the documents.
People Also Read: Use of Border Container in Flutter
Additionally, the Path Provide Flutter also allows developers to easily access the external storage directory on the device, which is useful for applications that need to save large files or backup data.
Table of Contents
Flutter Path Provider Method
You will get following method to access the device storage by using Path Provide Flutter.
- getApplicationDocumentsDirectory(): getApplicationDocumentsDirectory() is a method in Flutter that returns a directory where the app can store files that are meant to be persisted across app launches. These files will be deleted when the app is uninstalled.
- getTemporaryDirectory(): getTemporaryDirectory() is a method in Flutter that returns a directory where the app can store temporary files. These files may be deleted by the system at any time, so they should not be used for storing important data. This is typically used for caching files that can be regenerated if deleted.
- getExternalStorageDirectory(): getExternalStorageDirectory() is a method in Flutter that returns the primary external storage directory of the device. This directory is typically used to store files that are meant to be shared with other apps or persist across app launches. The returned path may not be accessible if external storage is not available, or the app does not have the necessary permissions to access it.
- getDownloadsDirectory(): getDownloadsDirectory() is a method in Flutter that returns the device’s download directory, where the user can typically find files that they have downloaded through the internet or other apps. This directory is often used by apps that need to download and store files such as images, videos, documents and more. It’s important to note that the returned path may not be accessible if the app does not have the necessary permissions to access it.
- getExternalCacheDirectories(): getExternalCacheDirectories() is a method in Flutter that returns a list of directories where an app can store cache files on external storage devices, such as an SD card. This method is useful for apps that need to store temporary data that can be regenerated if deleted. The method returns a list of directories, in case the device has multiple external storage options. It’s important to note that the returned paths may not be accessible if the external storage is not available, or the app does not have the necessary permissions to access it, and that the files in the cache may be deleted by the system at any time.
- getLibraryDirectory():
getLibraryDirectory()
is a method in the Android operating system that returns the path to the directory on the device where the application can place persistent files it owns. This directory is internal to the application and is not accessible to other applications (nor to the user). It is best suited for files that the user doesn’t need to directly interact with. - getApplicationSupportDirectory():
getApplicationSupportDirectory()
is a method in the iOS operating system that returns the path to the directory where an application can place files it needs to support its operation. This directory is internal to the application and is not visible to the user. It is best suited for files such as databases, user-generated content, and other application-specific files that the user doesn’t need to directly interact with. It is similar to Android’s getLibraryDirectory()
Path Provide Flutter
To use Path Provider in a Flutter project, you’ll first need to add it as a dependency in your pubspec.yaml
file:
dependencies:
path_provider: ^2.0.11
Example1: Get Temporary and Documents App Directory Path
You can then import the Path Provide Flutter package and use Path Provide Flutter in your code:
Now import the Path Provide Flutter package on your main.dart
import 'package:path_provider/path_provider.dart';
import 'dart:io';
Temporary Directory Path Code:
Directory tempDir = await getTemporaryDirectory();
temp_Path = tempDir.path;
print("temp_path: $temp_Path");
//output: /data/user/0/com.example.flutterserviceexample/cache
App Document Directory Path Code:
Directory appDir = await getApplicationDocumentsDirectory();
appPath = appDir.path;
print("app_path: $appPath");
//output: /data/user/0/com.example.flutterserviceexample/app_flutter
You can use these paths to read and write files in the flutter app.
Full Code:
import 'package:flutter/material.dart';
import 'package:path_provider/path_provider.dart';
import 'dart:io';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.lightBlue,
),
home: const MyHomePage(title: 'Flutter Service'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String temp_Path = "";
String appPath = "";
getPathLocation() async {
Directory tempDir = await getTemporaryDirectory();
temp_Path = tempDir.path;
print("temp_path: $temp_Path");
Directory appDir = await getApplicationDocumentsDirectory();
appPath = appDir.path;
print("app_path: $appPath");
}
@override
void initState() {
getPathLocation();
super.initState();
}
@override
void setState(VoidCallback fn) {
// TODO: implement setState
getPathLocation();
super.setState(fn);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
padding: EdgeInsets.all(10),
alignment: Alignment.center,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text("Temp Path: $temp_Path"),
Text("App Path: $appPath")
],
)));
}
}
Output
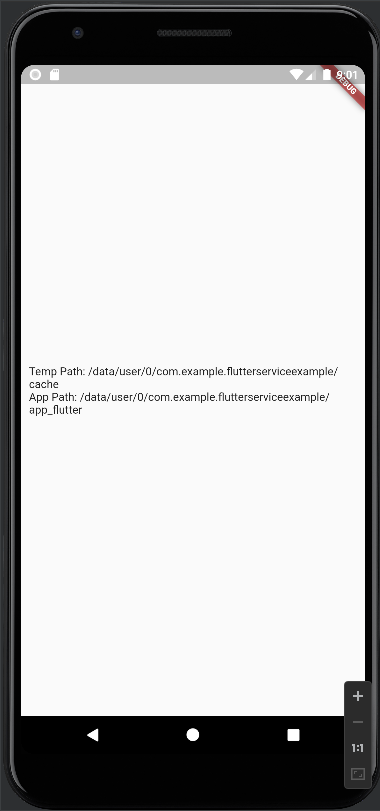
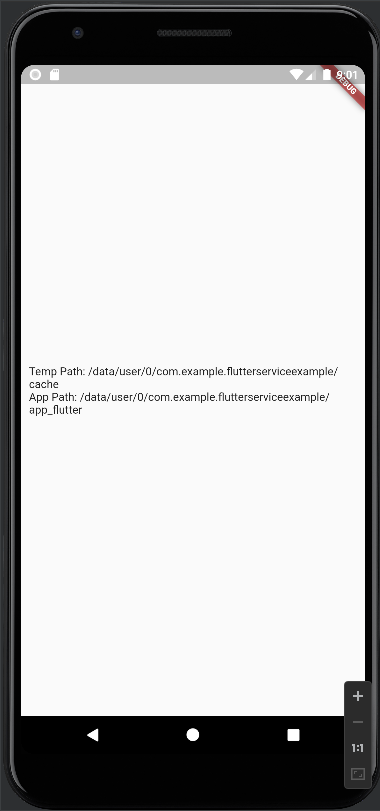
Example 2: Read & Write a Text File
At first add the Path Provide Flutter dependency on pubspec.yaml
dependencies:
path_provider: ^2.0.11
Now initialize the text editing controller and receive a text filed.
final textController = TextEditingController();
String text = "";
Now let’s create create 2 method named createFile and readFile.
Future<void> createFile(String text) async {
final directory = await getApplicationDocumentsDirectory();
//creates text_file in the provided path.
final file = File('${directory.path}/text_file.txt');
await file.writeAsString(text);
}
Future<void> readFile() async {
try {
final directory = await getApplicationDocumentsDirectory();
final file = File('${directory.path}/text_file.txt');
text = await file.readAsString();
} catch (e) {
print('exception');
}
}
We will create a text file named text_file.txt
Now when the task destroyed we want to remove the textcontroller dispose.
@override
void dispose() {
textController.dispose();
super.dispose();
}
Now add the button and textfield on build widget.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("Flutter Service")),
body: Column(
children: [
Padding(
padding: const EdgeInsets.symmetric(horizontal: 18.0, vertical: 10),
child: TextField(
textAlign: TextAlign.center, //input aligns to center
controller: textController, //assigns TextEditingController
),
),
const SizedBox(
height: 8,
),
ElevatedButton(
child: Text('Create File'),
onPressed: () => createFile(
textController.text), //calls createFile() when //button pressed
),
ElevatedButton(
onPressed: () async {
await readFile(); //calls readFile()
setState(() {}); //rebuilds the UI.
},
child: Text('Read File'),
),
const SizedBox(
height: 18,
),
if (text != null) Text('$text') //text set if it's not null.
],
),
);
}
Full Code is
import 'package:flutter/material.dart';
import 'package:path_provider/path_provider.dart';
import 'dart:io';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.lightBlue,
),
home: const MyHomePage(title: 'Flutter Service'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final textController = TextEditingController();
String text = "";
Future<void> createFile(String text) async {
//provides directory path.
final directory = await getApplicationDocumentsDirectory();
//creates text_file in the provided path.
final file = File('${directory.path}/text_file.txt');
await file.writeAsString(text);
}
Future<void> readFile() async {
try {
final directory = await getApplicationDocumentsDirectory();
final file = File('${directory.path}/text_file.txt');
text = await file.readAsString();
} catch (e) {
print('exception');
}
}
@override
void dispose() {
textController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("Flutter Service")),
body: Column(
children: [
Padding(
padding: const EdgeInsets.symmetric(horizontal: 18.0, vertical: 10),
child: TextField(
textAlign: TextAlign.center, //input aligns to center
controller: textController, //assigns TextEditingController
),
),
const SizedBox(
height: 8,
),
ElevatedButton(
child: Text('Create File'),
onPressed: () => createFile(
textController.text), //calls createFile() when //button pressed
),
ElevatedButton(
onPressed: () async {
await readFile(); //calls readFile()
setState(() {}); //rebuilds the UI.
},
child: Text('Read File'),
),
const SizedBox(
height: 18,
),
if (text != null) Text('$text') //text set if it's not null.
],
),
);
}
}
Output
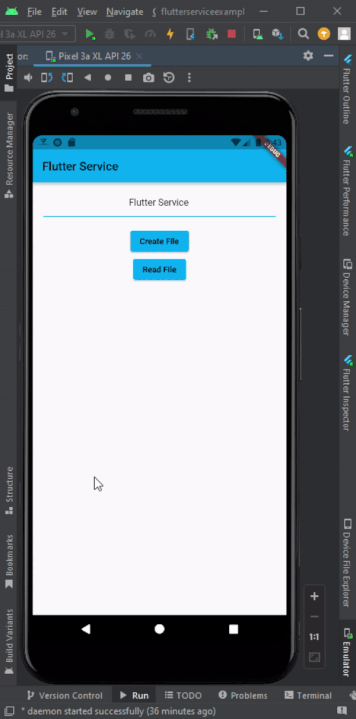
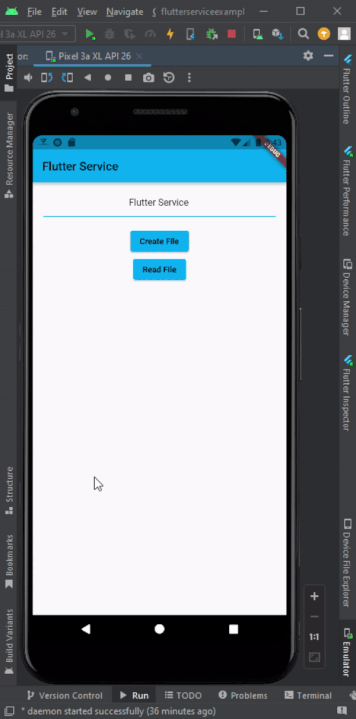
When click the create file, that time a file is created under the app storage. To view the file, Using Android Studio, View -> Tool Windows -> Device File Explorer.
Now go to this location: Go for this location /data/data/com.example.your_app/app_name/text_file.txt
This is the output.
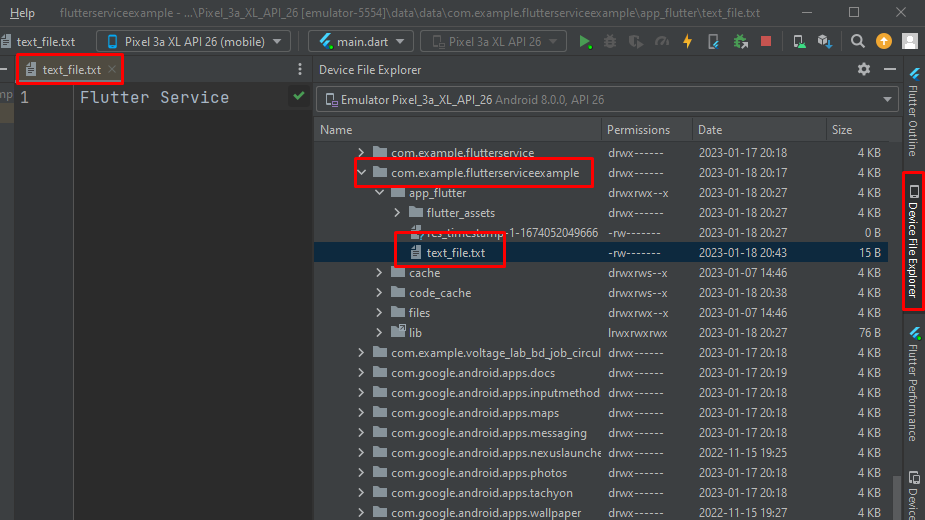
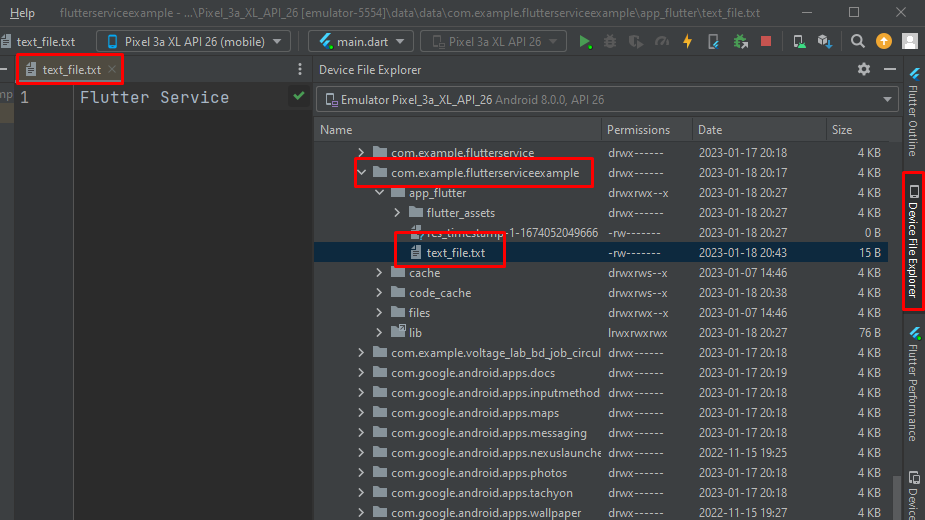
In conclusion, the Path Provide Flutter package is a useful for working with the file system on both Android and iOS devices. Path Provide Flutter allows developers to easily access and manipulate files and directories on the device. By using the provided methods, such as getApplicationDocumentsDirectory and getTemporaryDirectory, developers can easily access specific directories on the device.
Additionally, the package also allows for the creation, deletion, and manipulation of files and directories. Overall, the Path Provide Flutter package is a powerful package that can greatly simplify file system operations in Flutter apps.
Read More: