This tutorial gives an in-depth explanation of how to add a check box in flutter.
People, who read this article also read: Flutter SDK Installation on Windows, macOS, Linux
In this example, we’ll demonstrate how to add a checkbox in Flutter and make it switchable when the user clicks the label on the box. For any form, checkboxes are crucial elements. Here’s an illustration:
Table of Contents
Checkbox Addition in Flutter
bool? check1 = false; //true for checked checkbox, false for unchecked one
Checkbox( //only check box
value: check1, //unchecked
onChanged: (bool? value){
//value returned when checkbox is clicked
setState(() {
check1 = value;
});
}
)
Only a checkbox with an unchecked status is available here.
bool? check2 = true,;
CheckboxListTile( //checkbox positioned at right
value: check2,
onChanged: (bool? value) {
setState(() {
check2 = value;
});
},
title: Text("Do you really want to learn Flutter?"),
)
You will see a checkbox with a label here, but the checkbox’s status won’t change unless you click on it. Not clickable is the label.
How to Set the Left Checkbox on the CheckboxListTile
bool? check3 = false;
CheckboxListTile(
value: check3,
controlAffinity: ListTileControlAffinity.leading, //checkbox at left
onChanged: (bool? value) {
setState(() {
check3 = value;
});
},
title: Text("Do you really want to learn Flutter?"),
)
You will see a checkbox with a label that you can click here.
Checking whether a checkbox is selected:
bool? check1 = false;
Checkbox(
value: check1, //set variable for value
onChanged: (bool? value){
setState(() {
check1 = value;
});
}
)
if(check1!){
//checkbox is checked
}else{
//checkbox is not checked
}
Code to add a Check Box in Flutter
import 'package:flutter/material.dart';
void main(){
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
);
}
}
class Home extends StatefulWidget{
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
bool? check1 = false, check2 = true, check3 = false;
//true for checked checkbox, flase for unchecked one
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Checkbox in Flutter"),
backgroundColor: Colors.redAccent
),
body: Container(
padding: EdgeInsets.only(top:20, left:20, right:20),
alignment: Alignment.topLeft,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Checkbox( //only check box
value: check1, //unchecked
onChanged: (bool? value){
//value returned when checkbox is clicked
setState(() {
check1 = value;
});
}
),
CheckboxListTile( //checkbox positioned at right
value: check2,
onChanged: (bool? value) {
setState(() {
check2 = value;
});
},
title: Text("Do you really want to learn Flutter?"),
),
CheckboxListTile( //checkbox positioned at left
value: check3,
controlAffinity: ListTileControlAffinity.leading,
onChanged: (bool? value) {
setState(() {
check3 = value;
});
},
title: Text("Do you really want to learn Flutter?"),
),
],)
)
);
}
}
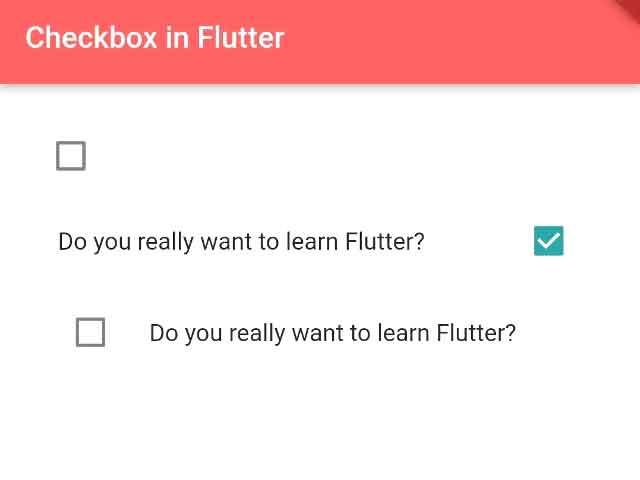
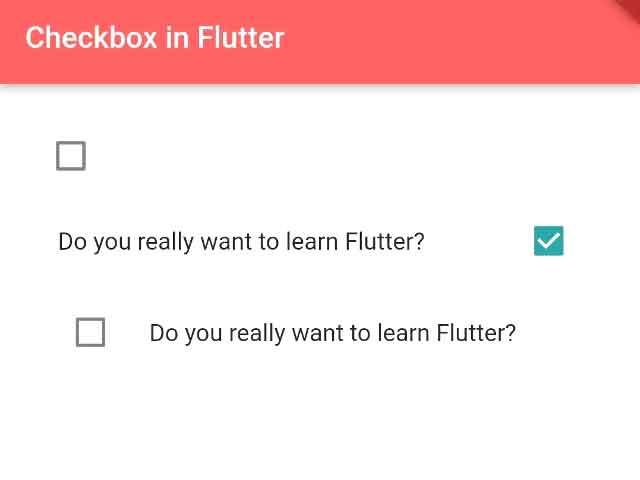
This will enable you to add a checkbox to the Flutter application.
People, who read this article also read: Flutter Liquid Swipe Animation
Conclusion
You’ve learned the basics of how to add a check box in Flutter.
Now, I’d like to hear from you: Do you know how to add a checkbox in flutter? Did you think it would be this easy?
Either way, let me know by leaving a quick comment below.
Check the following video for visual insight!