In this article, we will learn some easy tricks of how to add border to container in Flutter.
There could be a lot of Container widgets added while creating a Flutter app. However, on occasion, you might want to include a container with a border to create a box UI for things like buttons, keys, cards, etc. We’ll discover in this tutorial how to add a border to a container in Flutter.
Table of Contents
People, who read this article also read: Flutter Liquid Swipe Animation
Increase the container’s border
By including the BoxDecoration class in the Container’s decoration property, you can give it a border. This is the first of many ways in order to add border to container in Flutter.
Instructions for adding a border to a container in Flutter:
- First, head over to the Container where you want to add a border.
- Assign the BoxDecoration class and add the decoration parameter. Put the border parameter inside the BoxDecoration and set it to Border.all().
- Launch the app.
What your code should look like is as follows:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border.all(),
),
child: const Text(
"FlutterService",
style: TextStyle(fontSize: 34.0),
),
)
Border.all() creates a black border on all sides of a container. It is a very special type of constructor.
Output:
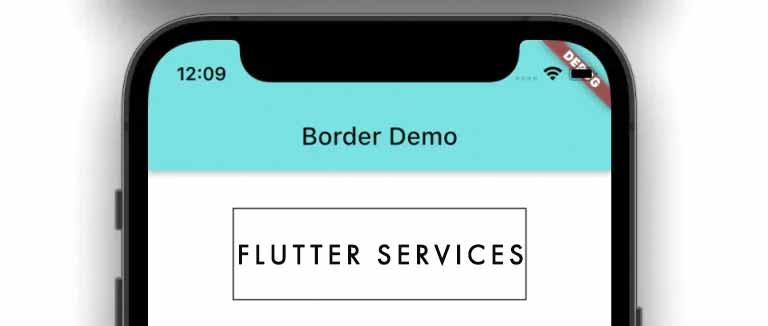
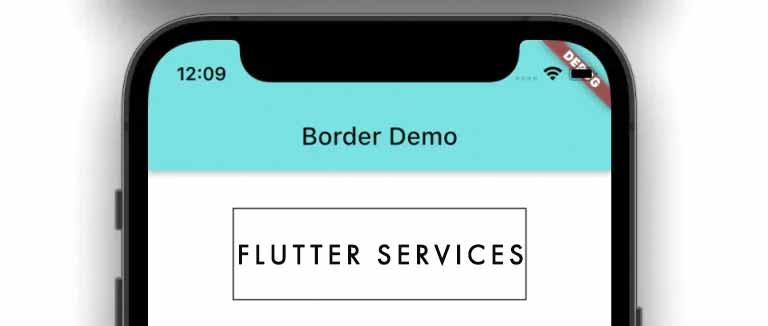
Here is an example of how the code is translated into the design:
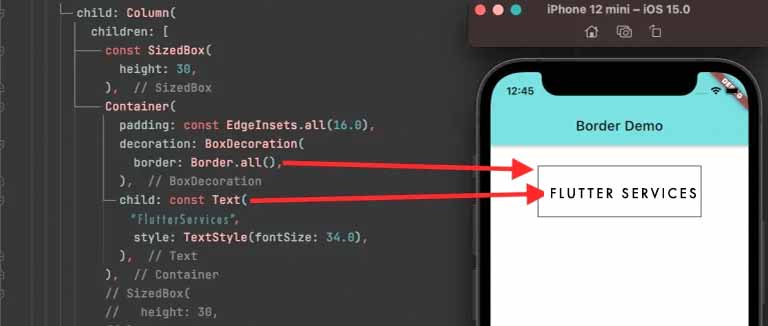
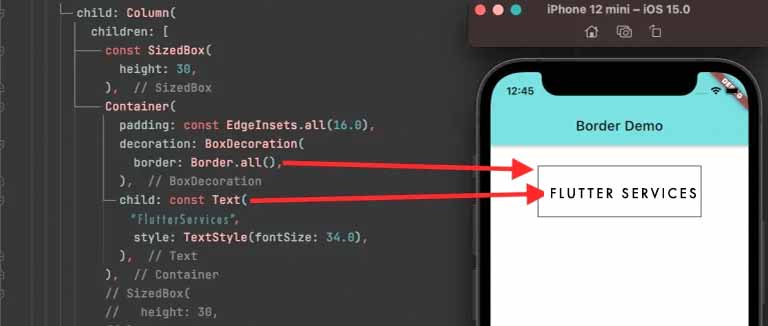
Border Width Adding
By default, the width of the border created by the Border.all() constructor is set to 1. To create a unique user interface, you might want to alter the border width and default value.
In order to give the container a wider border:
Adding the width parameter’s value inside the Border is all that is necessary. all constructor.
As for the code:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border.all(
width: 10,
),
),
child: const Text(
"FlutterService",
style: TextStyle(fontSize: 34.0),
),
)
Output:
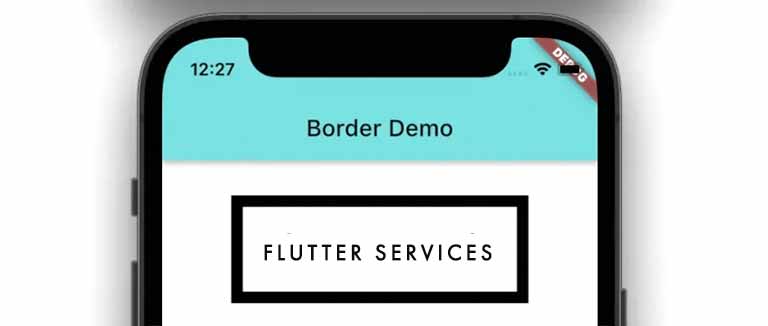
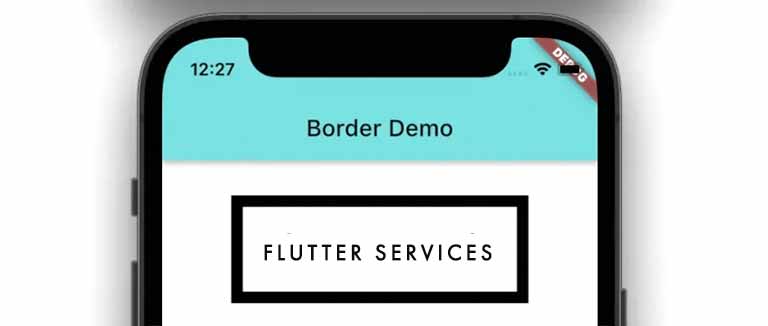
Border Color Modification
Black is added as the default color by the constructor for Border.all. Using the color property in the Border.all constructor, you can change the default color to any other color.
People, who read this article also read: Flutter SDK Installation on Windows, macOS, Linux
How to modify the container’s border color
Include your unique color in the color parameter for the Border.all.
What your code should look like is as follows:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border.all(width: 5, color: Colors.deepPurpleAccent),
),
child: const Text(
"FlutterService",
style: TextStyle(fontSize: 34.0),
),
)
Output:
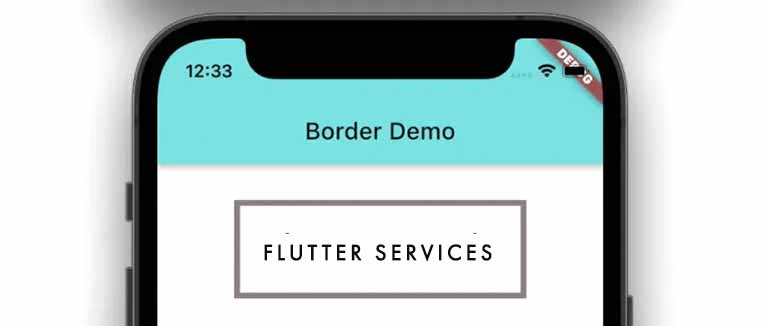
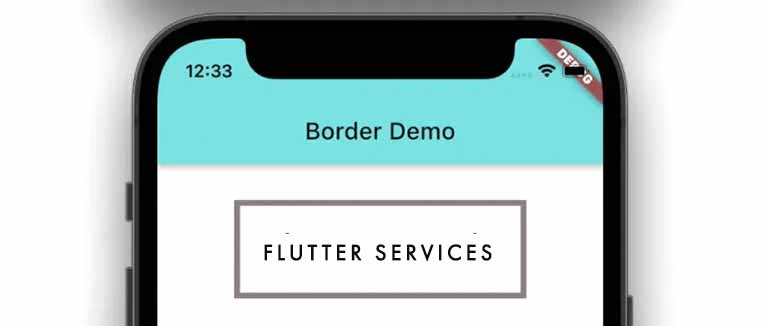
Constructing a Container with Border Radius | How to add Border to Container in Flutter
You might need to add rounded corners to the container’s corners to give the UI a button-like appearance. By giving the container’s border-radius, this can be accomplished.
The steps to adding border radius to a container are as follows:
- Visit the Container where you want to add a border radius in step 1.
- Adding the decoration parameter and assigning the BoxDecoration class are step two. The borderRadius parameter should be added inside the BoxDecoration and set to BorderRadius.all (Radius.circular(50)).
- Run the app in the third step.
Following is an example of good code:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border.all(width: 5, color: Colors.red),
borderRadius: BorderRadius.all(Radius.circular(50)),
),
child: const Text(
"FlutterService",
style: TextStyle(fontSize: 34.0),
),
)
Output:
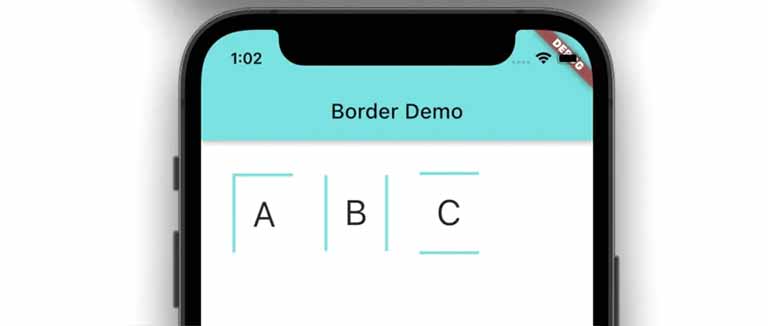
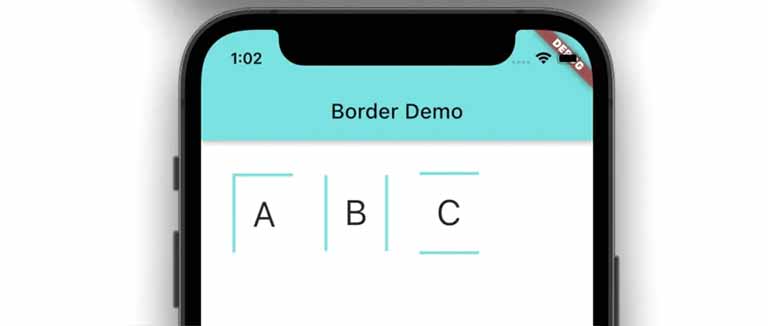
To Add Border to Container in Flutter on a Few Sides
You might occasionally want to add a border to just a few of the container’s sides, such as the left and right, top and bottom, only the bottom, etc.
How to increase the container’s border-radius
- Go to the Container first, and then choose which sides you want to have borders on.
- Assign the BoxDecoration class and add the decoration parameter. Add the border parameter to the BoxDecoration and assign the BorderSide class to any of the container’s four sides (left, top, right, and bottom).
- Run the app in the third step.
As for the code:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border(
top: BorderSide(
color: Color(0xff6ae792),
width: 3.0,
),
bottom: BorderSide(
color: Color(0xff6ae792),
width: 3.0,
),
),
),
child: const Text(
"C",
style: TextStyle(fontSize: 34.0),
),
)Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border(
top: BorderSide(
color: Color(0xff6ae792),
width: 3.0,
),
bottom: BorderSide(
color: Color(0xff6ae792),
width: 3.0,
),
),
),
child: const Text(
"C",
style: TextStyle(fontSize: 34.0),
),
)
Output:
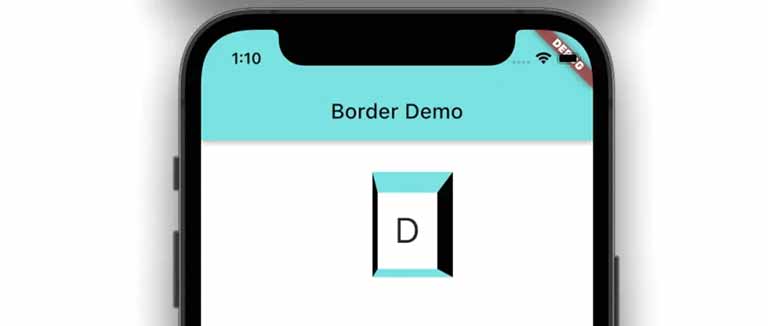
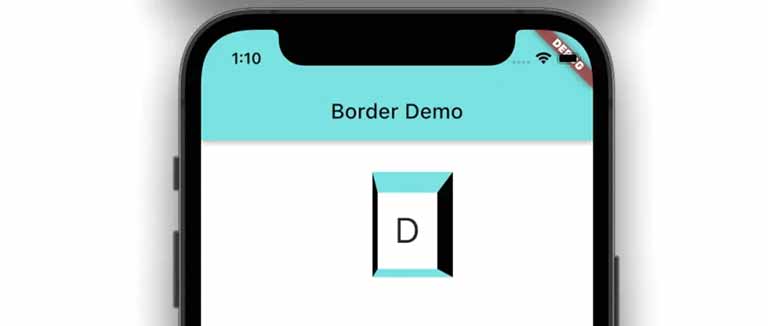
The code is as follows, and you can use the same method to adjust the container’s border on all sides:
Container(
padding: const EdgeInsets.all(16.0),
decoration: BoxDecoration(
border: Border(
left: BorderSide(
color: Colors.black,
width: 5.0,
),
right: BorderSide(
color: Colors.black,
width: 15.0,
),
top: BorderSide(
color: Color(0xff6ae792),
width: 20.0,
),
bottom: BorderSide(
color: Color(0xff6ae792),
width: 8.0,
),
),
),
child: const Text(
"D",
style: TextStyle(fontSize: 34.0),
),
)
Preview:
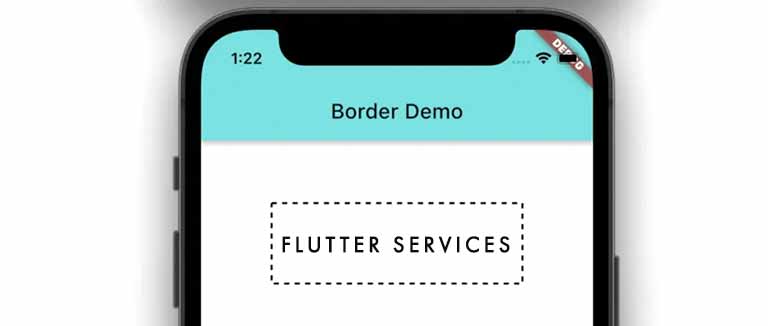
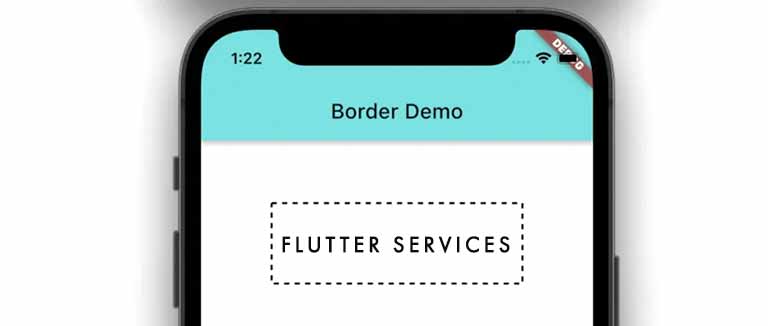
Add Border to Container in Flutter i.e. Dotted Border
Designing a UI with a dotted border may be necessary. For instance, a draggable area with a dotted border can be displayed during a drag-and-drop interaction. Let’s use dotted_border to add a dotted border to the container’s perimeter.
Follow these steps to give the container a dotted border:
- Open the pubspec.yaml file in step 1 and include the dotted_border package.
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.2
dotted_border: ^2.0.0+1
- The second step is to enclose your Container widget inside the DottedBorder widget.
- Add the strokeWidth, color, and dashPattern parameters in step 3.
- Run your app in step four.
As for the code:
DottedBorder(
color: Colors.black,
strokeWidth: 2,
dashPattern: [
5,
5,
],
child: Container(
padding: const EdgeInsets.all(
16.0),
child: const Text(
"FlutterService",
style: TextStyle(fontSize: 34.0),
),
),
)
Output:
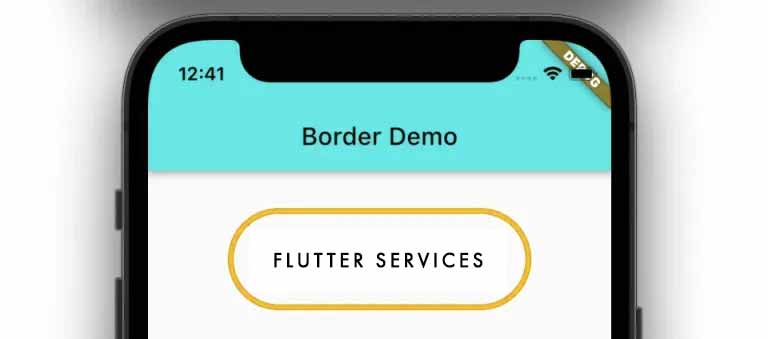
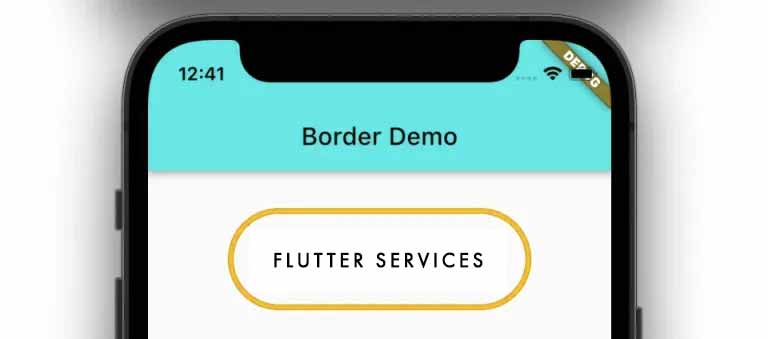
There you go. I appreciate you reading this.
Conclusion
With the help of real-world examples, we learned how to add border to container in Flutter in this tutorial. Additionally, we learned how to alter the container’s border and width and how to add a border radius before seeing how to add a dotted border.